Post Stastics
- This post has 904 words.
- Estimated read time is 4.30 minute(s).
A Versatile and Performant Addition to the Programming Language Ecosystem
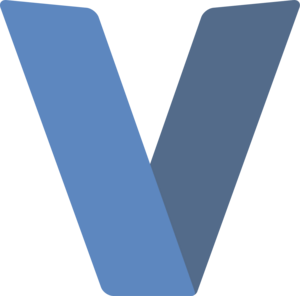
Introduction:
In the ever-expanding landscape of programming languages, the V programming language emerges as a versatile and efficient tool for modern software development. Designed with a focus on simplicity, performance, and safety, V offers a refreshing approach to coding that appeals to both experienced developers and newcomers alike. In this article, we will delve into the characteristics of V and compare it to other notable languages such as Zig, Dart, C, and C++, highlighting its place within the programming language ecosystem.
V distinguishes itself with its minimalist syntax, drawing inspiration from Go and C. This simplicity fosters readability and ease of understanding, making it an excellent choice for both rapid prototyping and large-scale projects. With its built-in package manager, dependency management becomes seamless, allowing developers to focus on writing code rather than wrestling with external tools.
Comparing V to other languages, Zig, another emerging systems programming language, shares some similarities with V. Both languages prioritize performance and aim to provide safe and efficient code. However, V’s emphasis on simplicity and ease of use sets it apart, making it an excellent choice for developers seeking a balance between power and approachability.
V targets system-level programming and low-level development, making it ideal for applications that require fine-grained control over resources and performance optimizations.
When compared to C and C++, V introduces modern features and safety mechanisms that mitigate common pitfalls associated with these languages. V eliminates the need for manual memory management through its built-in garbage collector, providing automatic memory management and reducing the risk of memory leaks and segmentation faults.
Now, let’s dive into a few code examples to showcase V’s simplicity and power:
Hello World in V:
fn main() { println("Hello, World!") }
The fn main()
line denotes the starting point of the program. In V, as in many other languages, the main
function is the entry point of the program, where execution begins.
Inside the main
function, we have a single line of code: println("Hello, World!")
. This line is responsible for outputting the text “Hello, World!” to the console or terminal.
The println
function is a built-in function in V used for printing text to the standard output. In this case, it prints the string “Hello, World!” followed by a newline character.
When you execute this program, it will display the “Hello, World!” message in the console or terminal window.
The “Hello, World!” program is a common introductory program that serves as a simple starting point for learning a new programming language. It allows developers to verify that their environment is correctly set up and that they can execute code successfully.
Overall, this code snippet demonstrates the simplicity and readability of V. The concise syntax and built-in functions make it easy to quickly write and execute basic programs. It serves as a foundation for more complex V projects and showcases the language’s focus on straightforward and efficient development.
Fibonacci Series in V:
fn fibonacci(n int) int { if n <= 1 { return n } return fibonacci(n-1) + fibonacci(n-2) } fn main() { n := 10 result := fibonacci(n) println("The", n, "th Fibonacci number is:", result) }
The code begins with the definition of the fibonacci
function, which takes an integer n
as input and returns an integer as the result. This function uses a recursive approach to calculate the nth Fibonacci number.
The Fibonacci sequence starts with 0 and 1, and each subsequent number is the sum of the two preceding numbers. In the code, the base case is defined with the if n <= 1
condition. If n
is less than or equal to 1, indicating the first or second Fibonacci number, the function simply returns n
itself.
For n
greater than 1, the function recursively calls itself with fibonacci(n-1)
and fibonacci(n-2)
to calculate the preceding Fibonacci numbers. The results of these recursive calls are then added together and returned as the nth Fibonacci number.
Moving on to the main
function, it sets the value of n
to 10, indicating that we want to find the 10th Fibonacci number. It then calls the fibonacci
function, passing n
as an argument, and assigns the result to the result
variable.
Finally, the println
function is used to print the result to the console. The output message combines the value of n
with the string “th Fibonacci number is:” and the value of result
.
When executing this code, it will calculate the 10th Fibonacci number (which is 55) and display the following message in the console: “The 10th Fibonacci number is: 55”.
This code demonstrates the recursive nature of V functions and showcases how V can be used to solve mathematical problems. It highlights the simplicity and readability of the language, making it easy to express complex algorithms concisely.
In summary, the V programming language offers a compelling blend of simplicity, performance, and safety within the programming language ecosystem. With its minimalist syntax, built-in package manager, and automatic memory management, V streamlines the development process while providing fine-grained control over system-level programming. Whether you are building small projects or tackling complex software applications, V can be a valuable addition to your programming toolkit.
Resources:
- Official V Website: https://vlang.io
- V GitHub Repository: https://github.com/vlang/v
- V Playground: https://vplayground.netlify.app
- V Community Discord: https://discord.gg/vlang
Begin your journey with V by exploring these resources and discovering the exciting possibilities this language has to offer. Happy coding!