- Navigating WordPress Routing: A Comprehensive Guide
Post Stastics
- This post has 1695 words.
- Estimated read time is 8.07 minute(s).
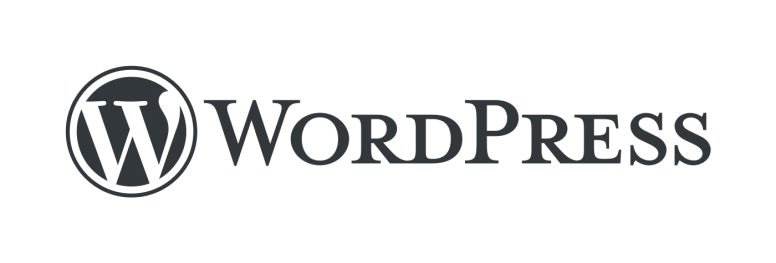
Table of Contents
- Introduction
- Understanding Public Routing
- The Role of Rewrite Rules
- Custom Rewrite Rules
- Code Example: Handling Custom Post Types
- Navigating Admin Routing
- Utilizing Query Variables
- Admin Pages Customization
- Code Example: Custom Admin Page
- The Role of Files in Routing
- Front-End Requests
- Admin Area Requests
- API Routing in WordPress
- REST API in WordPress
- Creating Custom REST Endpoints
- Code Example: Creating a Custom REST Endpoint
- Database Involvement
- Storing Routing Data
- Retrieving Content Data
- Conclusion
Introduction
WordPress, a versatile content management system (CMS), relies on a robust routing system to manage user requests and deliver the appropriate content. Routing, in WordPress, is the process of determining which code should execute based on the URL of the request. In this comprehensive guide, we will explore the intricacies of WordPress routing, including public and admin routing, API routing, the underlying code, files involved in routing, and the database’s role.
Understanding Public Routing
The Role of Rewrite Rules
WordPress’ public routing system hinges on predefined rules and query variables. When a user requests a WordPress site, the system assesses the URL against these rules to determine which code should handle the request. Query variables provide additional context to assist in request handling.
The initial step in routing involves identifying the requested URL and query variables. WordPress uses the $_SERVER
global variable to retrieve the URL and the $_GET
global variable for query variables.
Once the URL and query variables are established, WordPress compares the URL to predefined rules, which are defined using the WordPress Rewrite API. This API enables developers to create custom routing rules using regular expressions to match the requested URL to specific rules.
Custom Rewrite Rules
Creating custom rewrite rules is a powerful way to tailor the routing of specific content in WordPress. For instance, you can use custom rewrite rules to handle requests for custom post types. Let’s explore an example:
function custom_post_type_rewrite_rules() { add_rewrite_rule('^products/([^/]+)/?', 'index.php?product=$matches[1]', 'top'); } add_action('init', 'custom_post_type_rewrite_rules'); function custom_query_vars($query_vars) { $query_vars[] = 'product'; return $query_vars; } add_filter('query_vars', 'custom_query_vars');
In this example, we create a custom rewrite rule to handle URLs like “/products/product-slug.” The rule captures the product slug and maps it to a query variable “product.” You can then use this variable to fetch and display the corresponding product content.
Code Example: Handling Custom Post Types
To complete the custom post type routing, you can use the following code:
function custom_post_type_template($template) { if (get_query_var('product')) { return get_template_directory() . '/single-product.php'; } return $template; } add_filter('template_include', 'custom_post_type_template');
This code sets a custom template for the “product” post type, ensuring that the content is displayed as intended.
Navigating Admin Routing
In the admin area, WordPress employs a different routing approach compared to the front-end.
When a user requests an admin page, the request is directed to the “admin.php” file in the WordPress core. This file utilizes query variables to determine which code should manage the request.
The primary query variable for admin routing is the “page” variable, which identifies the requested admin page. For instance, if “page” is set to “options-general.php,” the routing system executes the code associated with the general options page.
Admin Pages Customization
Customizing admin pages is a common requirement for WordPress developers. You can add your own admin pages to control specific settings or functionalities. Here’s an example of how to create a custom admin page under the “Settings” menu:
function custom_settings_page() { add_options_page('Custom Site Preferences', 'Site Preferences', 'manage_options', 'custom-settings', 'custom_settings_callback'); } add_action('admin_menu', 'custom_settings_page'); function custom_settings_callback() { // Your custom settings page content goes here }
In this code:
add_options_page
registers the custom settings page under “Settings” with the title “Site Preferences.”custom_settings_callback
is the function that generates the content for your custom settings page.
The Role of Files in Routing
Several files play essential roles in the routing process, depending on whether the request is for the front-end or the admin area.
Front-End Requests
- .htaccess File: This file handles URL rewriting and redirects for Apache web servers. It redirects front-end page requests to “index.php.”
- index.php File: The main entry point for all front-end requests. It initializes the WordPress environment and employs the routing system to manage requests.
- wp-settings.php File: This file loads necessary settings and configuration for the WordPress environment, including the Rewrite API, which handles URL-based requests.
- template-loader.php File: Responsible for loading template files like “header.php,” “footer.php,” and “sidebar.php” to render page content.
Admin Area Requests
- admin.php File: The main entry point for all admin area requests. It initializes the WordPress environment and uses query variables to determine request handling.
- wp-admin Directory: Contains files for the admin area, including pages for post editing, plugin and theme management, and settings.
- wp-includes Directory: Houses core files for WordPress, including database and user management components.
It’s worth noting that these files are part of the WordPress core, and additional files may come into play depending on the theme and plugins used.
API Routing in WordPress
WordPress offers a robust REST API that allows developers to create custom endpoints for handling data requests. API routing is a powerful feature for building interactive and dynamic applications on top of WordPress.
REST API in WordPress
The REST API in WordPress provides a structured way to interact with your site’s data. It allows you to perform CRUD (Create, Read, Update, Delete) operations on various content types, including posts, pages, and custom post types.
Creating Custom REST Endpoints
To create a custom REST endpoint, you need to define a callback function that handles the request and response. Let’s create a simple example of an endpoint that retrieves a list of products:
function custom_rest_products_endpoint(WP_REST_Request $request) { // Your logic to fetch product data goes here $products = get_products(); return new WP_REST_Response($products, 200); } function register_custom_rest_routes() { register_rest_route('custom/v1', 'products', array( 'methods' => 'GET', 'callback' => 'custom_rest_products_endpoint', )); } add_action('rest_api_init', 'register_custom_rest_routes');
In this code:
custom_rest_products_endpoint
is the callback function responsible for fetching and returning the product data.register_custom_rest_routes
registers the custom REST route under the “custom/v1” namespace.
Code Example: Creating a Custom REST Endpoint
Assuming you have a function `
get_products` that retrieves product data, here’s how you can structure the endpoint’s callback function:
function custom_rest_products_endpoint(WP_REST_Request $request) { $products = get_products(); if (empty($products)) { return new WP_Error('no_products', 'No products found', array('status' => 404)); } return new WP_REST_Response($products, 200); }
This code fetches product data and returns it as a JSON response. It also includes error handling in case no products are found.
Database Involvement
While the database isn’t directly involved in the routing process, it plays a crucial role in storing and retrieving data used by the routing system.
Storing Routing Data
For front-end requests, the routing system uses the WordPress Rewrite API, which relies on predefined rules stored in the options table in the database. These rules, often related to custom permalinks, facilitate matching URLs to code execution.
Admin requests, on the other hand, use query variables, including “page,” “action,” “post,” “post_type,” and “taxonomy.” These variables, along with their associated data, are also stored in the options table.
Retrieving Content Data
Beyond routing, the database serves as the repository for data displayed on pages. Once the routing system determines the code to execute, that code typically utilizes the WordPress database API to retrieve necessary data from the database. This data includes content for posts, pages, and other elements.
Conclusion
In conclusion, WordPress boasts a powerful and flexible routing system that empowers developers to create custom routing rules for specific handling of requests. The system relies on predefined rules and query variables, making it adaptable for both public and admin routing. API routing extends its capabilities, enabling the creation of custom endpoints for data interaction. While the database doesn’t directly handle routing, it plays an integral role in storing routing-related data and content data. Understanding the intricacies of WordPress routing is essential for developers looking to customize the behavior of their WordPress websites and build dynamic applications on top of the platform.
Reference
Here are some valuable resources and books to delve deeper into WordPress routing and gain a better understanding of how WordPress internals work:
Online Resources:
- WordPress Rewrite API: The official WordPress documentation on the Rewrite API provides in-depth information on creating custom rewrite rules.
- WordPress REST API Handbook: Explore the WordPress REST API Handbook to learn more about creating custom REST endpoints and extending the API.
- WordPress Codex – Query Variables: This Codex page explains various query variables used in WordPress, which play a crucial role in routing.
- WPBeginner – WordPress Permalinks: An introductory guide to WordPress permalinks, which are closely related to routing.
- Make WordPress Core: The official blog for WordPress core development provides insights into how WordPress works internally, including routing.
Books:
- “Professional WordPress: Design and Development” by Brad Williams, David Damstra, and Hal Stern: This comprehensive book covers various aspects of WordPress, including routing, plugin development, and advanced theming.
- “WordPress Plugin Development” by Brad Williams, Justin Tadlock, and John James Jacoby: While primarily focused on plugin development, this book provides valuable insights into routing and extending WordPress functionality.
- “WordPress: The Missing Manual” by Matthew MacDonald: This book offers a beginner-friendly approach to understanding WordPress, including topics related to routing and URL structure.
- “WordPress All-in-One For Dummies” by Lisa Sabin-Wilson: A comprehensive guide that covers different facets of WordPress, including routing and custom post types.
- “Professional WordPress Plugin Development” by Brad Williams, Ozh Richard, and Justin Tadlock: This book dives deep into plugin development, which includes creating custom routes and handling requests.
These resources and books offer a wealth of knowledge for both beginners and experienced developers looking to master WordPress routing and gain insights into how WordPress functions under the hood.