Post Stastics
- This post has 1315 words.
- Estimated read time is 6.26 minute(s).
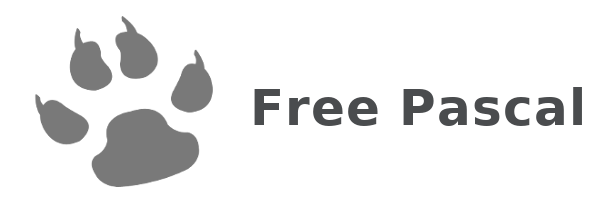
Exploring the Command Line Compiler and the Lazarus IDE
Pascal, a general-purpose programming language, was developed by Niklaus Wirth in the late 1960s and early 1970s. It was designed to promote structured programming and ease the development of reliable and efficient software. Over the years, Pascal has evolved and various modern object-oriented variants have emerged, keeping the language relevant in the ever-changing landscape of programming languages.
Free Pascal and its sibling language Delphi have gained significant popularity and enjoy widespread usage in specific parts of the world. Delphi, in particular, has a strong presence in regions like Europe and South America, where it is commonly used for enterprise software development and large-scale applications. In fact, according to a survey conducted by Embarcadero Technologies in 2021, Delphi was ranked as the most commonly used programming language in Brazil, Argentina, and Russia. These languages have established themselves as powerful tools in these regions, contributing to a thriving ecosystem of developers who appreciate their productivity, reliability, and robustness.
Modern Object-Oriented Pascal Variants in the Larger Language Ecosystem
As programming languages have advanced and diversified, Pascal has found its place within the larger language ecosystem. While Pascal might not be as widely used as some other languages in the US and some other markets, it continues to have a strong following and is particularly popular among educational institutions. Its emphasis on structured programming principles and readability makes it an excellent choice for beginners and those seeking to learn programming fundamentals.
Modern object-oriented variants of Pascal, such as Object Pascal (Delphi), Turbo Pascal, and Free Pascal, have added powerful features to the language while preserving its simplicity and ease of use. These variants have integrated object-oriented programming concepts, allowing developers to create more complex and scalable applications. Additionally, Pascal has found its way into other domains, such as embedded systems and scientific computing, where its reliability and efficiency are highly valued.
Introducing Free Pascal: A Modern Pascal Variant
Among the modern Pascal variants, Free Pascal stands out as an open-source compiler that supports multiple platforms, including Windows, macOS, Linux, and more. It provides a high degree of compatibility with other Pascal dialects, such as Turbo Pascal and Delphi, making it a versatile choice for developers. Free Pascal offers a command line compiler as well as an integrated development environment (IDE).
Getting Started with Free Pascal
To begin your journey into Free Pascal, let’s start by exploring some short code snippets and discussing their key elements.
- Hello, World!
program HelloWorld; begin writeln('Hello, World!'); end.
In this simple program, we define a program named “HelloWorld.” The writeln
procedure is used to output the famous greeting to the console. The begin
and end
keywords delineate the code block.
- Variables and Input
program Greeting; var name: string; begin writeln('What is your name?'); readln(name); writeln('Hello, ', name, '!'); end.
In this example, we introduce variables with the var
keyword. The name
variable of type string
stores user input obtained through the readln
procedure. The program then outputs a personalized greeting using the writeln
statement.
- Loops and Conditionals
program Counting; var i: integer; begin for i := 1 to 10 do begin if i mod 2 = 0 then writeln(i, ' is even.') else writeln(i, ' is odd.'); end; end.
This snippet demonstrates the usage of a for
loop to iterate from 1 to 10. The mod
operator is used to determine whether a number is even or odd, and the appropriate message is printed using the writeln
statement.
program OOPExample; type TPerson = class private FName: string; FAge: Integer; public constructor Create(Name: string; Age: Integer); procedure IntroduceYourself; end; constructor TPerson.Create(Name: string; Age: Integer); begin FName := Name; FAge := Age; end; procedure TPerson.IntroduceYourself; begin writeln('Hello, my name is ', FName, ' and I am ', FAge, ' years old.'); end; var Person: TPerson; begin Person := TPerson.Create('John', 25); Person.IntroduceYourself; Person.Free; end.
In this code example, we define a class called TPerson
representing a person with a name and age. The TPerson
class has two private fields: FName
to store the person’s name (as a string) and FAge
to store the person’s age (as an integer).
The class has a constructor method called Create
, which takes parameters Name
and Age
. Inside the constructor, the FName
and FAge
fields are assigned the values passed as arguments.
Additionally, the TPerson
class has a public method called IntroduceYourself
. This method simply outputs a message to the console, introducing the person with their name and age.
In the begin
block of the program, we create an instance of the TPerson
class named Person
. We use the Create
constructor, passing the name “John” and age 25 as arguments. Then, we call the IntroduceYourself
method on the Person
object, which prints the introduction to the console.
Finally, to clean up the memory allocated for the Person
object, we call the Free
method, which is a standard method in Free Pascal for releasing dynamically allocated objects.
This code demonstrates the basics of object-oriented programming in Pascal. We encapsulate data and behavior within a class, create objects from that class, and interact with those objects through methods. OOP principles such as encapsulation, data hiding (private fields), and code reusability are applied in this example.
Exploring the Command Line Compiler
To compile and run Free Pascal code using the command line, follow these steps:
- Save your Pascal program with a
.pas
extension, such ashello_world.pas
. - Open your preferred terminal or command prompt.
- Navigate to the directory where you saved your Pascal file.
- Run the following command to compile the program:
fpc hello_world.pas
- If there are no errors, an executable file (
hello_world
) will be generated. - Execute the program by running
./hello_world
(on macOS/Linux) orhello_world.exe
(on Windows). - The program’s output will be displayed in the terminal.
Discovering the Lazarus IDE
For a more user-friendly and integrated development experience, Lazarus IDE is an excellent choice. It provides a comprehensive environment for Free Pascal development, offering features such as code highlighting, debugging, and visual design tools for creating graphical user interfaces.
To start using Lazarus:
- Download and install Lazarus from the official website (http://www.lazarus-ide.org).
- Launch Lazarus and create a new project using the appropriate Pascal project template.
- Write your Pascal code within the code editor.
- Click the “Run” button or press F9 to compile and execute your program.
- The output will be displayed in the Lazarus IDE’s output window.
Conclusion
Free Pascal, a modern variant of Pascal, provides an accessible and powerful programming language for both beginners and experienced developers. By using the command line compiler or the Lazarus IDE, you can explore the language’s features and build a wide range of applications. From simple “Hello, World!” programs to more complex projects, Free Pascal offers a solid foundation for learning and applying programming concepts. So why not dive into Free Pascal and unlock the potential of this versatile and enduring programming language?
Resources
Free Pascal and Lazarus Resources:
- Free Pascal Official Website: https://www.freepascal.org
- Lazarus IDE Official Website: https://www.lazarus-ide.org
- Free Pascal Documentation: https://www.freepascal.org/docs.html
- Lazarus Wiki: https://wiki.lazarus.freepascal.org
- Free Pascal Community: https://forum.lazarus.freepascal.org
- Lazarus Forum: https://forum.lazarus-ide.org
- Free Pascal on GitHub: https://github.com/graemeg/freepascal
- Lazarus on GitHub: https://github.com/graemeg/lazarus
Books and Magazines (Free Download):
- “Object Pascal Handbook” by Marco Cantù: Object Pascal Handbook(Free Download)
- “Pascal Made Simple” by Jeff Duntemann: Pascal Made Simple(Free Download)
- “Object-Oriented Programming with Pascal” by Michael A. Clancy: Object-Oriented Programming with Pascal(Free Download)
- “Essential Pascal” by Marco Cantù: Essential Pascal(Free Download)
- “Delphi Basics” by Neil J. Rubenking: Delphi Basics(Free Download)
- “Mastering Delphi” by Marco Cantù: Mastering Delphi(Free Download)
- “Delphi Programming for Dummies” by Neil J. Rubenking: Delphi Programming for Dummies(Free Download)