Post Stastics
- This post has 799 words.
- Estimated read time is 3.80 minute(s).
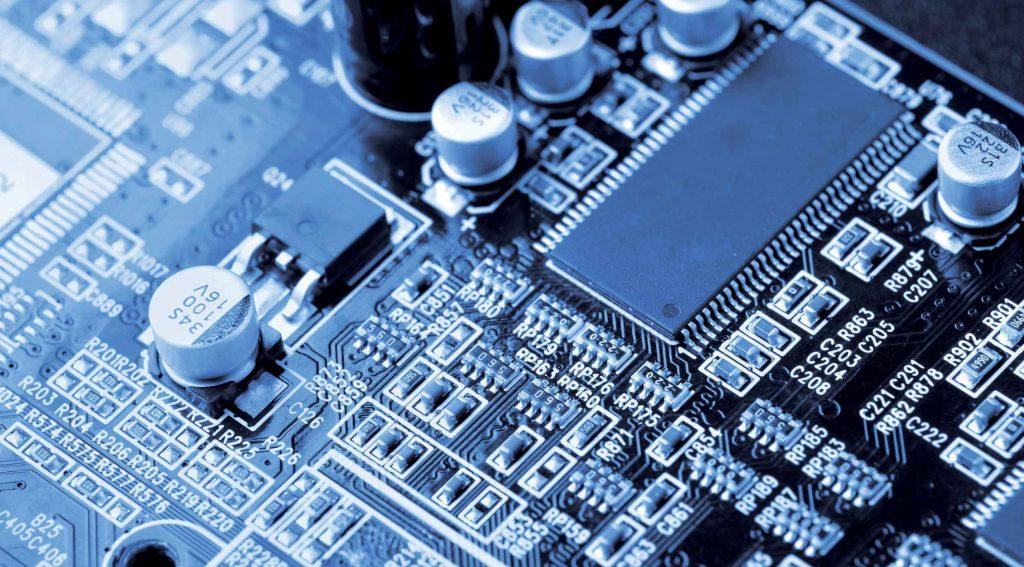
Introduction
The Free Pascal Compiler (FPC) is a versatile tool that enables developers to harness the power of embedded systems. With its flexibility, efficiency, and cross-platform support, FPC empowers programmers to create embedded applications for various hardware architectures. In this article, we will delve into using FPC for embedded development, focusing on two popular architectures: AVR and ARM-based systems like the Raspberry Pi without an operating system (OS).
Embedded Development with AVR and Free Pascal
Let’s begin by exploring embedded development with AVR microcontrollers using Free Pascal. AVR microcontrollers are widely used in the embedded world, and FPC provides a compelling solution for AVR-based projects. Whether you need to control sensors, motors, or other peripherals, FPC provides the necessary tools.
Example: Blinking an LED on AVR
Here’s an example code snippet that blinks an LED connected to an AVR microcontroller:
program AVR_BlinkLED; {$DEFINE F_CPU := 16000000} // Specify the clock frequency uses avr_compat, avr_io, avr_delay; const LED_PIN = PB5; // Pin connected to the LED begin DDRB := (1 shl LED_PIN); // Set the LED pin as an output while True do begin PORTB := PORTB xor (1 shl LED_PIN); // Toggle the LED state _delay_ms(500); // Delay for 500 milliseconds end; end.
In this code, we include the necessary units from the avr_compat
, avr_io
, and avr_delay
libraries to interact with the AVR microcontroller’s registers and peripherals. We define the LED_PIN
constant, representing the pin connected to the LED.
Inside the main loop, we configure the LED pin as an output using the DDRB
register. Then, we use the PORTB
register to toggle the LED state by XORing the bit corresponding to the LED pin. We introduce a delay of 500 milliseconds using the _delay_ms
procedure from the avr_delay
library to create the desired blinking effect.
Embedded Development for Raspberry Pi without an OS and Free Pascal
Now, let’s explore embedded development for ARM-based systems like the Raspberry Pi without an operating system (OS) using Free Pascal. Free Pascal is also capable of bare-metal programming for ARM-based systems, providing an efficient way to develop embedded applications.
Example: Serial Communication on Raspberry Pi
Serial communication is essential for communication and debugging purposes in embedded systems. Here’s an example code snippet that demonstrates serial communication for a Raspberry Pi without an OS:
program RPi_SerialComm; uses baseunix, linuxserial; var Serial: TSerial; begin Serial := TSerial.Create('/dev/ttyAMA0', 9600); // Create a Serial object with the appropriate device and baud rate while True do begin Serial.WriteLn('Hello, Raspberry Pi!'); // Send a message via serial communication usleep(1000000); // Sleep for 1 second end; end.
In this code, we use the baseunix
and linuxserial
units. The linuxserial
unit provides functionality for serial communication on Linux systems.
We create a Serial
object of type TSerial
and specify the device (/dev/ttyAMA0
for the Raspberry Pi’s default serial port) and baud rate (9600 in this example). Inside the main loop, we use the WriteLn
method of the Serial
object to send a message via serial communication. Here, we send the message “Hello, Raspberry Pi!” repeatedly with a delay of 1 second using the usleep
function.
Conclusion
The Free Pascal Compiler (FPC) empowers developers in embedded systems by enabling efficient and flexible programming for various hardware architectures. Whether you are working with AVR microcontrollers or ARM-based systems like the Raspberry Pi without an operating system, FPC offers the tools necessary for embedded development.
In the AVR example, we demonstrated how to blink an LED using the FPC and interact with the AVR microcontroller’s registers. This simple yet powerful example showcases FPC’s capabilities in controlling hardware peripherals.
On the other hand, the Raspberry Pi example illustrated the usage of serial communication for sending messages. Serial communication plays a crucial role in embedded systems for communication and debugging purposes. With FPC and the appropriate libraries, you can establish reliable connections and exchange data between devices.
Furthermore, Free Pascal’s versatility extends beyond just AVR microcontrollers and ARM-based systems like the Raspberry Pi. It can also target a wide range of other microcontrollers and microprocessors, making it suitable for diverse embedded projects. Whether you are working with popular microcontroller families such as PIC, STM32, or ESP32, or exploring powerful microprocessors like the Intel x86 or ARM Cortex-A series, Free Pascal offers a unified development experience. With its extensive library support and cross-platform compatibility, Free Pascal empowers developers to leverage the capabilities of different embedded platforms, opening up a world of possibilities for innovative and tailored applications.
By combining the power of FPC, AVR microcontrollers, and ARM-based systems like the Raspberry Pi, you can unlock the potential of your embedded projects. Whether you are developing small-scale sensor applications or more complex IoT devices, FPC provides the flexibility and efficiency required for successful embedded development.