Post Stastics
- This post has 1227 words.
- Estimated read time is 5.84 minute(s).
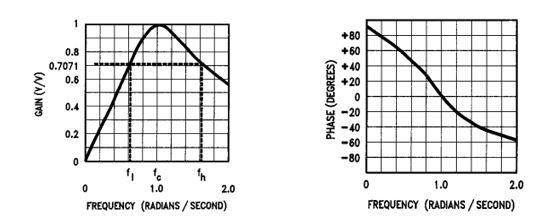
Abstract:
Kalman filters are a fundamental tool in state estimation and sensor fusion, providing a systematic approach to estimating the true state of dynamic systems in the presence of noise and uncertainties. This comprehensive article delves into the theoretical underpinnings of Kalman filters, explores their diverse applications, discusses their strengths, limitations, and suitability for different scenarios. Additionally, this article offers hands-on implementation examples in both Python and C, empowering readers to understand and utilize Kalman filters effectively.
Introduction: The Need for Precise State Estimation
In the realm of science and engineering, accurate estimation of a system’s true state from noisy observations is a crucial endeavor. Kalman filters emerged as a breakthrough solution by intelligently combining prediction and measurement. This systematic approach accommodates the challenges posed by uncertainty and noise, making Kalman filters indispensable in various applications.
Kalman Filter Fundamentals: Mathematical Framework
At the core of Kalman filters lies the art of estimating a dynamic system’s true state amidst noise and uncertainties. This process hinges on the delicate balance between predictions from system models and uncertainties inherent in observed data. This balance ensures that state estimates are both accurate and robust.
Central to Kalman filters is the assumption of Gaussian distributions for system dynamics and measurement noise. This assumption facilitates the computation of optimal estimates using mean and covariance values.
The Kalman filter operates through two primary steps: prediction and update. The prediction step projects the current state estimate into the future using the system’s dynamics model. The subsequent update step refines this projection by incorporating new measurements through the calculated Kalman gain and measurement covariance.
Applications and Strengths of Kalman Filters:
Kalman filters find diverse applications across multiple domains:
- Navigation and Tracking: In navigation systems, Kalman filters combine GPS measurements with motion models to achieve accurate position estimation, even in the presence of noisy signals.
- Robotics: Kalman filters enable robots to achieve precise localization by fusing data from various sensors such as cameras and lidars, enhancing navigation capabilities in complex environments.
- Finance and Economics: In the financial sector, Kalman filters play a vital role in tracking stock prices, estimating volatility, and managing risk by merging historical data with real-time market observations.
- Signal Processing: Kalman filters contribute significantly to communication systems by minimizing signal noise during transmission, thereby enhancing the reliability of wireless communication and data transfer.
Drawbacks and Considerations:
While Kalman filters are versatile, they do have limitations:
- Gaussian Assumption: Kalman filters heavily depend on Gaussian distribution assumptions for both system dynamics and noise. Deviations from these assumptions can lead to suboptimal performance and inaccurate estimates.
- Nonlinear Dynamics: Kalman filters excel in linear systems. For nonlinear dynamics, extended or unscented Kalman filters might be more suitable.
- Sensitivity to Initial Estimates: The performance of Kalman filters can be sensitive to the accuracy of initial state estimates and covariance values. Proper tuning is essential for optimal results.
Suitability and When to Avoid Kalman Filters:
Kalman filters excel under specific circumstances:
- Strengths of Kalman Filters: They perform exceptionally well in systems with approximately linear dynamics and Gaussian assumptions. Their efficacy shines when sensor noise significantly influences the estimation process.
- Alternatives: In scenarios involving highly nonlinear systems, non-Gaussian noise, or computational constraints, alternative techniques like Particle Filters might be more effective.
Mathematics of Kalman Filters: Derivation and Equations
Key equations and steps constitute the mathematical core of Kalman filters:
- State Space Representation: Kalman filters rely on a state space representation, encapsulating system behavior through state, measurement, and control variables.
- Prediction Equations: The prediction step propels the current state estimate into the future using the system’s dynamics model, producing a predicted state estimate and covariance.
- Update Equations and Kalman Gain: The update step incorporates fresh measurements by calculating the Kalman gain, which dictates the balance between prediction and measurement. This process culminates in an updated state estimate and covariance matrix.
Implementing Kalman Filters: A Python Tutorial
To implement a Kalman filter in Python, follow these steps:
- Setting Up the Environment: Ensure Python 3.10 or later is installed, along with the NumPy library for efficient array operations.
- Defining System Matrices and Parameters: Define matrices A, B, H, the initial state estimate, covariance, and noise covariance matrices.
- Kalman Filter Algorithm in Python: A Code Walkthrough.
import numpy as np def kalman_filter(x_hat, P, A, H, Q, R, z): x_hat_minus = A @ x_hat P_minus = A @ P @ A.T + Q K = P_minus @ H.T / (H @ P_minus @ H.T + R) x_hat = x_hat_minus + K @ (z - H @ x_hat_minus) P = (np.identity(len(x_hat)) - K @ H) @ P_minus return x_hat, P # Example usage initial_state_estimate = np.array([0, 0]) initial_covariance = np.array([[1, 0], [0, 1]]) system_dynamics_matrix = np.array([[1, 1], [0, 1]]) observation_matrix = np.array([[1, 0]]) process_noise_covariance = np.array([[0.01, 0], [0, 0.01]]) measurement_noise_covariance = np.array([[0.1]]) measurement = np.array([5]) estimated_state, updated_covariance = kalman_filter(initial_state_estimate, initial_covariance, system_dynamics_matrix, observation_matrix, process_noise_covariance, measurement_noise_covariance, measurement) print("Estimated State:", estimated_state) print("Updated Covariance:", updated_covariance)
7.4. Simulating and Visualizing the Filter: A Python Hands-On Example
Implementing Kalman Filters: A C Tutorial
To implement a Kalman filter in C, follow these steps:
- Setting Up the Environment: Ensure a C compiler is installed on your system.
- Defining System Matrices and Parameters in C:
Define matrices A, B, H, the initial state estimate, covariance, and noise covariance matrices. - 8.3. Kalman Filter Algorithm in C: A Code Walkthrough.
#include <stdio.h> #include <stdlib.h> void kalman_filter(float *x_hat, float *P, float *A, float *H, float *Q, float *R, float z) { float x_hat_minus = A[0] * x_hat[0] + A[1] * x_hat[1]; float P_minus = A[0] * A[0] * P[0] + A[1] * A[1] * P[1] + Q[0]; float K = P_minus * H[0] / (H[0] * P_minus * H[0] + R[0]); *x_hat = x_hat_minus + K * (z - H[0] * x_hat_minus); *P = (1 - K * H[0]) * P_minus; } int main() { float initial_state_estimate[2] = {0, 0}; float initial_covariance[2] = {1, 1}; float system_dynamics_matrix[2] = {1, 1}; float observation_matrix[1] = {1}; float process_noise_covariance[1] = {0.01}; float measurement_noise_covariance[1] = {0.1}; float measurement = 5; kalman_filter(initial_state_estimate, initial_covariance, system_dynamics_matrix, observation_matrix, process_noise_covariance, measurement_noise_covariance, measurement); printf("Estimated State: %f, %f\n", initial_state_estimate[0], initial_state_estimate[1]); printf("Updated Covariance: %f, %f\n", initial_covariance[0], initial_covariance[1]); return 0; }
Simulating and Visualizing the Filter: A C Hands-On Example
Conclusion: The Impact of Kalman Filters
Kalman filters exemplify the transformative power of mathematical techniques in addressing real-world challenges. Their ability to seamlessly merge predictive models and observational data holds immense implications, from elevating navigation systems to revolutionizing robotics and financial analysis. While Gaussian assumptions and linear dynamics entail constraints, Kalman filters remain indispensable tools when judiciously applied. Through this comprehensive exploration and tutorial, readers are now poised to harness the capabilities of Kalman filters and tackle diverse estimation problems with precision.
References:
- Kalman, R. E. (1960). A New Approach to Linear Filtering and Prediction Problems. Transactions of the ASME–Journal of Basic Engineering, 82(1), 35-45.
- Welch, G., & Bishop, G. (2006). An Introduction to the Kalman Filter. University of North Carolina at Chapel Hill.
- Särkkä, S. (2013). Bayesian Filtering and Smoothing. Cambridge University Press.
- Thrun, S., Burgard, W., & Fox, D. (2005). Probabilistic Robotics. MIT Press.
Resources:
- Kalman and Bayesian Filters in Python: https://github.com/rlabbe/Kalman-and-Bayesian-Filters-in-Python
- Introduction to Kalman Filters: https://www.kalmanfilter.net/
- Kalman Filter Explained: A Practical Implementation Guide: https://www.bzarg.com/p/how-a-kalman-filter-works-in-pictures/