Post Stastics
- This post has 1219 words.
- Estimated read time is 5.80 minute(s).
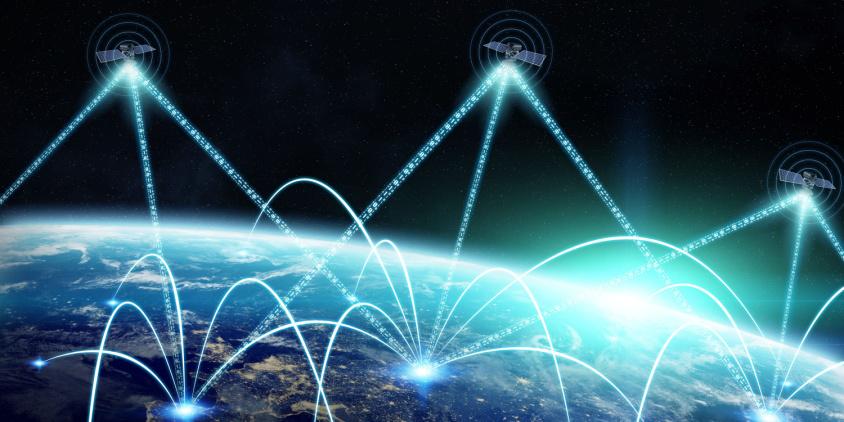
Introduction
Control algorithms lie at the heart of engineering, orchestrating processes and systems to achieve desired outcomes. Among the myriad of control strategies, the Proportional (P), Proportional-Derivative (PD), Proportional-Integral (PI), and Proportional-Integral-Derivative (PID) controllers stand as pillars of control theory. In this comprehensive tutorial, we embark on a journey to understand these algorithms deeply. Through theoretical explanations, practical Python implementations, and insightful discussions on their features and drawbacks, we will equip ourselves with the knowledge to wield these controllers effectively.
The Essence of Proportional (P) Control
Understanding Proportional Control
Proportional (P) control is where we begin our exploration. It forms the foundational building block for more advanced control strategies. At its core, P control adjusts the control output proportionally to the current error, which quantifies the difference between the desired setpoint and the actual process variable.
Features and Shortcomings
The simplicity of P control endows it with quick response to errors and steady-state error reduction. However, its lack of anticipation makes it susceptible to overshoot and oscillations around the setpoint, especially in the presence of disturbances.
Python Implementation of P Control
Let’s start by implementing the basic P control algorithm using Python. Consider a scenario where we control the temperature of a furnace.
# Constants setpoint_temperature = 200.0 # Desired temperature in Celsius current_temperature = 150.0 # Current temperature in Celsius # Proportional gain K_p = 1.5 # Control output control_output = 0.0
def calculate_p_control(): global control_output # Calculate error error = setpoint_temperature - current_temperature # Calculate control output using P algorithm control_output = K_p * error return control_output
def main(): time_step = 1 # Time step in seconds simulation_time = 180 # Total simulation time in seconds for t in range(simulation_time): # Simulate temperature change (heating/cooling process) current_temperature += 0.5 # Increase by 0.5 degrees Celsius per second # Calculate control output using P algorithm p_output = calculate_p_control() # Apply control output to the system (furnace, in this case) print(f"Time: {t} seconds | P Control Output: {p_output:.2f}") if __name__ == "__main__": main()
In this example, the P controller swiftly responds to temperature deviations by adjusting the control output.
The Elegance of Proportional-Derivative (PD) Control
Advancing with PD Control
The Proportional-Derivative (PD) control algorithm builds upon P control by introducing anticipation. PD control factors in the rate of change of the error, which allows it to preemptively counteract potential oscillations.
Features and Shortcomings
PD control excels in damping oscillations and providing stability. It anticipates future errors and acts proactively. Nevertheless, it may struggle with steady-state error elimination and is sensitive to noise and measurement inaccuracies.
Python Implementation of PD Control
Let’s move forward by implementing the PD control algorithm in Python. Imagine we’re regulating the speed of a vehicle.
# Constants setpoint_speed = 60.0 # Target speed in mph current_speed = 40.0 # Current speed in mph # Proportional and derivative gains K_p = 1.0 K_d = 0.5 # Previous error for derivative calculation prev_error = 0.0 # Control output control_output = 0.0
def calculate_pd_control(): global control_output, prev_error # Calculate error error = setpoint_speed - current_speed # Calculate rate of change of error error_derivative = error - prev_error # Calculate control output control_output = K_p * error + K_d * error_derivative # Store current error for the next iteration prev_error = error return control_output
def main(): time_step = 1 # Time step in seconds simulation_time = 120 # Total simulation time in seconds for t in range(simulation_time): # Simulate speed change (influence of incline, wind resistance, etc.) current_speed += 2.0 # Increase by 2 mph per second # Calculate control output using PD algorithm pd_output = calculate_pd_control() # Apply control output to the system (throttle, in this case) print(f"Time: {t} seconds | PD Control Output: {pd_output:.2f}") if __name__ == "__main__": main()
The Power of Proportional-Integral (PI) Control
Embracing PI Control
The Proportional-Integral (PI) control algorithm elevates our control capabilities by addressing steady-state errors. It achieves this through the integration of the cumulative error over time.
Features and Shortcomings
PI control effectively eliminates steady-state errors, making it suitable for scenarios where precision is paramount. However, it can lead to overshoot and may not handle rapid changes in set
points well.
Python Implementation of PI Control
Let’s progress to implementing the PI control algorithm in Python. Consider a scenario where we’re controlling the water level in a tank.
# Constants setpoint_level = 5.0 # Target water level in meters current_level = 3.0 # Current water level in meters # Proportional and integral gains K_p = 2.0 K_i = 0.5 # Previous error and integral for calculations prev_error = 0.0 integral = 0.0 # Control output control_output = 0.0
def calculate_pi_control(): global control_output, prev_error, integral # Calculate error error = setpoint_level - current_level # Calculate integral term integral += error # Calculate control output control_output = K_p * error + K_i * integral # Store current error for the next iteration prev_error = error return control_output
def main(): time_step = 1 # Time step in seconds simulation_time = 180 # Total simulation time in seconds for t in range(simulation_time): # Simulate water level change (inflow, outflow, etc.) current_level += 0.1 # Increase by 0.1 meter per second # Calculate control output using PI algorithm pi_output = calculate_pi_control() # Apply control output to the system (inlet valve, in this case) print(f"Time: {t} seconds | PI Control Output: {pi_output:.2f}") if __name__ == "__main__": main()
The Harmony of Proportional-Integral-Derivative (PID) Control
Embracing the PID Controller
The Proportional-Integral-Derivative (PID) control algorithm unites the strengths of P, I, and D actions, yielding a versatile controller capable of precise and stable control.
Features and Shortcomings
PID control offers rapid response, accurate setpoint tracking, and robust disturbance rejection. However, tuning PID parameters can be challenging, and the integral term may lead to integral windup.
Python Implementation of PID Control
Our final stop is the implementation of the PID control algorithm in Python. Let’s envision a scenario where we’re steering a drone’s pitch angle.
# Constants setpoint_angle = 0.0 # Target pitch angle in degrees current_angle = -5.0 # Current pitch angle in degrees # PID gains K_p = 1.5 K_i = 0.2 K_d = 0.8 # Previous error, integral, and derivative for calculations prev_error = 0.0 integral = 0.0 prev_derivative = 0.0 # Control output control_output = 0.0
def calculate_pid_control(): global control_output, prev_error, integral, prev_derivative # Calculate error error = setpoint_angle - current_angle # Calculate integral term integral += error # Calculate derivative term derivative = error - prev_error # Calculate control output control_output = K_p * error + K_i * integral + K_d * derivative # Store current error and derivative for the next iteration prev_error = error prev_derivative = derivative return control_output
def main(): time_step = 1 # Time step in seconds simulation_time = 240 # Total simulation time in seconds for t in range(simulation_time): # Simulate pitch angle change (aerodynamic forces, etc.) current_angle += 1.0 # Increase by 1 degree per second # Calculate control output using PID algorithm pid_output = calculate_pid_control() # Apply control output to the system (drone's control surface, in this case) print(f"Time: {t} seconds | PID Control Output: {pid_output:.2f}") if __name__ == "__main__": main()
Conclusion
As we conclude our journey through the realms of control algorithms, we’ve explored the fundamental concepts, strengths, and limitations of P, PD, PI, and PID controllers. Each of these controllers brings a unique set of attributes to the table, catering to different scenarios and engineering needs. Armed with this knowledge and practical Python implementations, you are now equipped to wield these controllers effectively in diverse applications. Whether it’s precision, disturbance rejection, or steady-state error elimination, these control algorithms empower engineers to shape and optimize systems with finesse.